PowerShell for Beginners – An Introduction to the Basics
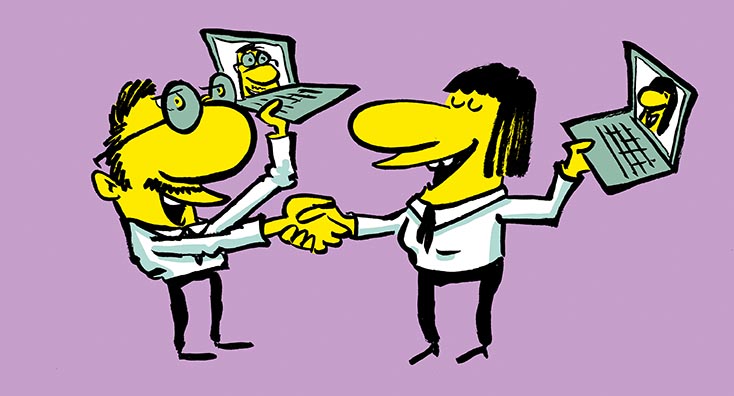
What is PowerShell?
In the modern world, everyone is striving for automation. For an administrator, performing the same repetitive tasks can become monotonous, which not only reduces efficiency but can also lead to errors.
PowerShell is an interactive command-line tool that allows you to automate these mundane tasks. You can run programs known as a “script (saved as a .ps1 file),” which contains several cmdlets for the task in question.
What is a Cmdlet?
A cmdlet is simply a command with which you can perform an action. The two most useful cmdlets that everyone should know are:
- Get-Command
- Get Help
With the ‘Get-Command’ cmdlet , you can find all available cmdlets, even if you don’t know the exact cmdlet. For example, you want to restart a service from PowerShell, but you don’t know the cmdlet. However, you can assume it contains the word “service.”
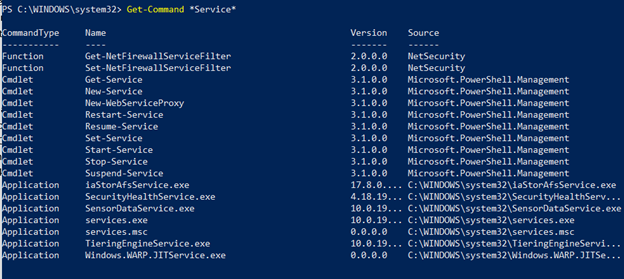
In the screenshot below, you have found all the available cmdlets that contain the word ‘service’ and thus, you have found the cmdlet to restart a service.
But how can you use this cmdlet? You can find more information about it using the ‘Get-Help’ cmdlet.
This provides basic information about the cmdlet and the parameters that can be used in it.
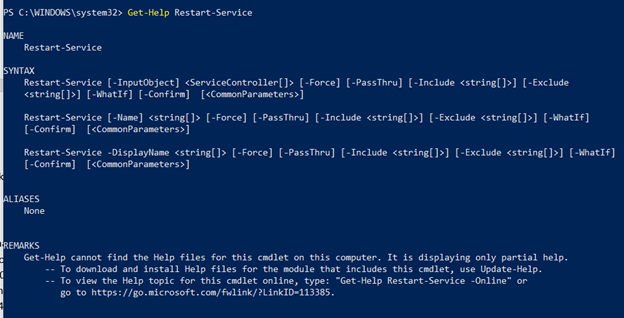
If you want to find the MS article on a cmdlet, just add the ‘-Online’ parameter at the end and it will open the MS page in the browser.
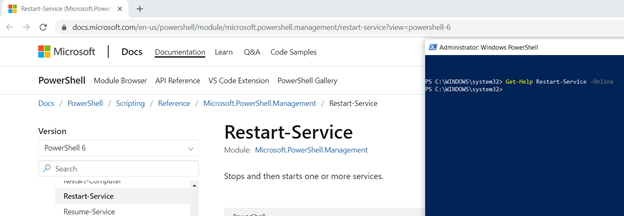
PowerShell vs. PowerShell ISE
Now you know how to run a program or cmdlet in PowerShell, but where to write a program? You can do this in PowerShell ISE.
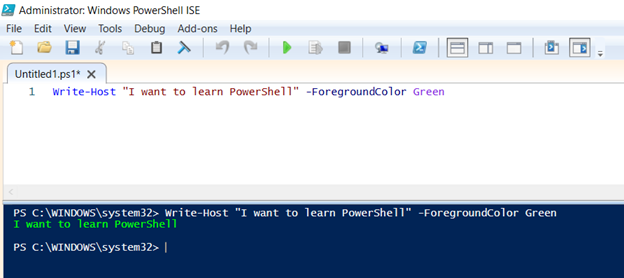
Variable
Any character preceded by the ‘$’ symbol becomes a variable. A variable is used when you need to store a value so that it can be used later in the script.
You have 2 values ’10’ and ‘4’ and you need to perform 3 functions on these values like addition, subtraction and multiplication. So there are 2 ways to do this task
a) 10+4, 10-4, 10*4
b) $a = 10
$b = 4
$a + $b, $a – $b, $a * $b
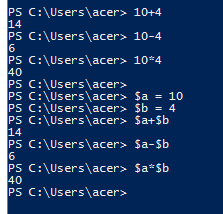
Data Types in PowerShell
There are different data types in PowerShell, including integers, floating-point values, strings, Booleans, and DateTime values, which are relevant to what you use in your daily life. The GetType method returns the current data type of the given variable.
Variables can be converted from one type to another by explicit conversion.
- Integer: It is of the “whole number” type. Any decimal value is rounded.
- Floating Point: The value is of decimal type.
- String: As the name suggests, it is used to store letters and characters.
- Boolean: This value can be either True or False. If it exists, then it is True, otherwise it will be False.
- DateTime: As the name suggests, this is a date and time type.
Arrays
Arrays are most commonly used to contain a list of values, such as a list of usernames or cities. PowerShell arrays can be defined by enclosing a list of items in parentheses and preceding it with the ‘@’ symbol. For example,
$nameArray = @(“John”,”Joe”,”Mary”)
Elements of an array can be accessed using their numeric index, starting with 0, in square brackets, as follows: $nameArray[0]. For example,
$nameArray[0] will be the first value in the array, i.e. “John”.
$nameArray[1] will be the second value in the array, i.e. “Joe”.
In simple terms, a table is like a column in Excel containing the same type of data.
Name |
John |
Joe |
Mary |
Exercise 1 : Create tables 1 and 2 as shown below. The third table should show the sum of each corresponding value in tables 1 and 2.
Array1 | Array2 | Array3 | ||
1 | 4 | 5 | ||
2 | 5 | 7 | ||
3 | 6 | 9 |
Hash table
A more advanced form of array, called a “hashtable,” is assigned with wavy parentheses preceded by the “@” sign. While arrays are typically (but not always) used to hold similar data, hash tables are better suited for related (rather than similar) data. Individual elements of a hash table are named rather than being assigned a numerical index, e.g.
$user=@{FirstName=”John”; LastName=”Smith”; MiddleInitial=”J”; Age=40}
The elements of a hash table can be easily accessed using the variable and key name, e.g.
$user.LastName will return the value “Smith”.
To easily understand hashtables, you can relate them with the following example.
In the example below, you have a table where the first column contains the “FirstName”, the second column contains the “LastName”, the third column contains the “MiddleInitial” and the fourth column contains the “Age”.
FirstName | LastName | MiddleInitial | Age |
John | Smith | D | 40 |
Joe | Parker | L | 32 |
Gary | Smith | N | 25 |
Now, if you store the above array in a hash table “$user”, then to call the first column you will need to run the command “$user.FirstName”, which will list all the first names in column 1.
Practice 2 : Create 2 hashtables as below and the third hashtable should be calculated as (DaysWorked * SalaryPerDay).
Hashtable 1 | Hashtable 2 | Hashtable 3 | |||||
Name | DaysWorked | Name | SalaryPerDay | Name | Salary | ||
John | 12 | John | 100 | John | 1200 | ||
Joe | 20 | Joe | 120 | Joe | 2400 | ||
Mary | 18 | Mary | 150 | Mary | 2700 |